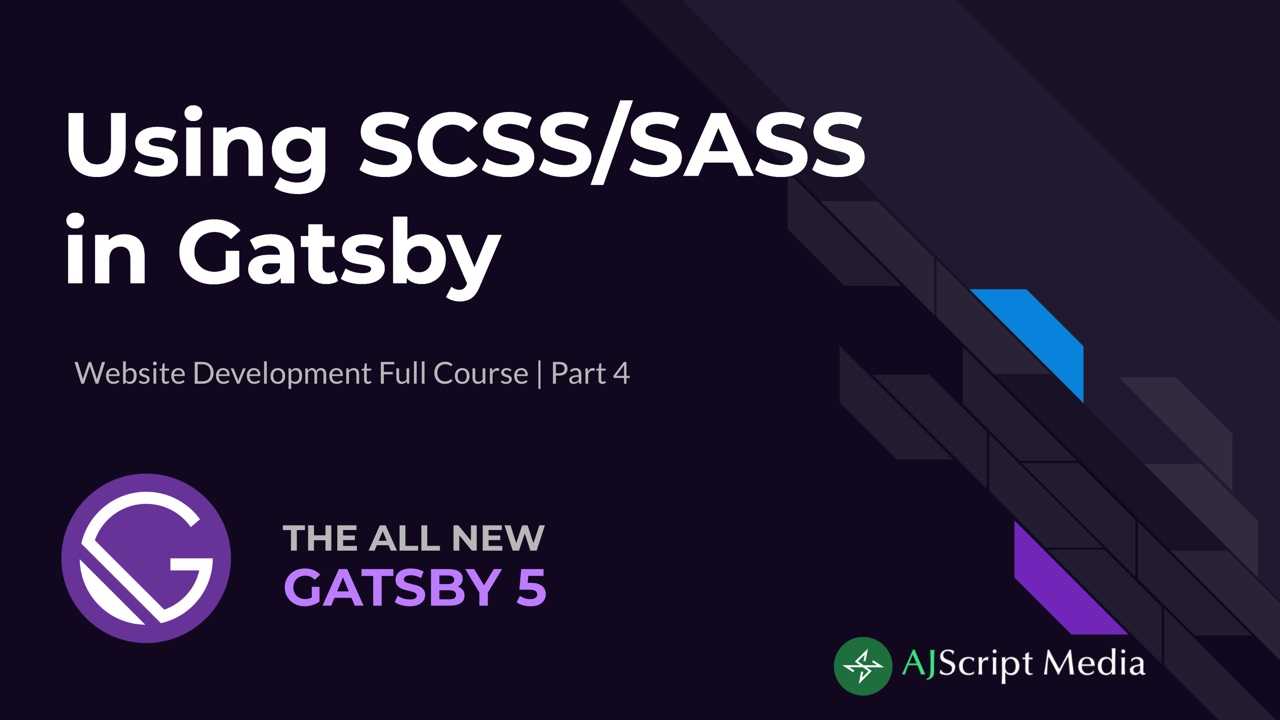
- /
- Gatsby/
- How to Use SCSS or SASS in Gatsby JS
In this tutorial, we will be discussing what is SCSS or SASS, why use it, how to install, discuss 7-1 pattern and finally we will be implementing SCSS to homepage hero image cover.
What is SCSS and SASS?
Open SCSSSCSS stands for Sassy Cascading Stylesheet or Sassy CSS while Open SASSSASS stands for Syntactically Awesome Style Sheet. The difference between SCSS and SASS is that SASS is the preprocessor scripting language while SCSS is the syntax used to write SASS. SCSS is a way to write all CSS valid syntax but with more advance functionalities. From the creator of SASS, they refer to it as "CSS with superpowers".
While SCSS syntax use traditional CSS syntax using curly brackets and semi colons, SASS uses indentions and newline. SCSS file extension is .scss and SASS file extension is .sass but both syntax will be compiled as a CSS file.
SASS Syntax:
SCSS Syntax:
Why use SASS?
In todays modern technology, SASS is used to make large website styling easier to manage. Traditional CSS will require a lot of code and hard to maintain specially if you're working with two or more developers.
With the advantage of SCSS, you can do a lot of functionalities like:
- Creating variables
- Calculations with operators like (+ , - , * , %)
- Mixin which is like creating a function that you can call and use with parameters. It makes code DRY (Don't Repeat Yourself)
- Nesting multiple selectors so that you don't need to copy every time you want to add styles to children elements
- Import partial SCSS file which allows you to separate your styles into different folders as per your needs
- Inheritance which allows you to copy shared css properties to different selectors and able to extend with new styles.
How to install SASS in Gatsby JS?
Now that we have learn what is SASS, it's time to implement to our Gatsby site. In order to use SASS, we need to install two node packages which are Open sasssass and Open gatsby-plugin-sassgatsby-plugin-sass.
Install needed node packages
Open your project using Open Visual Studio CodeVisual Studio Code and add new terminal by going to VS Code menu bar -> Terminal -> New Terminal. Copy the code below and paste it on terminal and hit enter. Installation might take some time depending on your internet connection.
Add SASS to Gatsby plugins
Once installation is done, we need to include it to Gatsby plugin before we can use it. Under gatsby-config.js, add gatsby-plugin-sass.
How to implement SASS or SCSS to Gatsby
In Gatsby, we can use both syntax but in this tutorial we will be using SCSS syntax. Under src folder, create a new folder and name it as "scss". Inside this folder, create a new file main.scss. This will be the main file which will store all our styles using import. Copy the code below and paste it to main.scss.
There are several ways we can use SASS in Gatsby. We can use it inside a component, use it in CSS Module or use it in gatsby-browser.js. In this tutorail, we will use it in Open gatsby-browser.jsgatsby-browser.js so let's create that file under root folder and copy the code below to import main.scss we created.
In order to check if SCSS is working, let's run Gatsby using this command in terminal gatsby-develop. Once development bundle is done, open your browser and go to http://localhost:8000/. You should see that the background color turns to red.
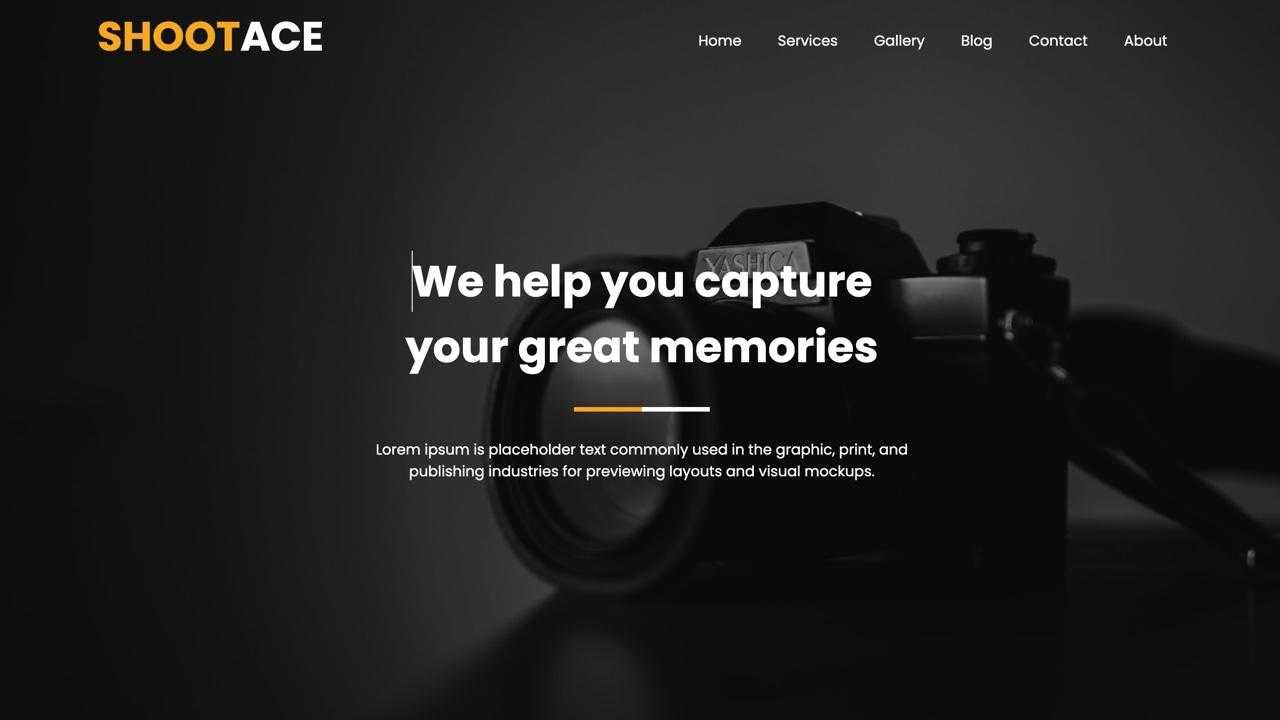
SCSS File Structure using 7-1 Pattern
Now we are ready to define our file structure. Inside scss folder, let's create different folders by using Open 7-1 Pattern7-1 Pattern which is good especially for bigger projects.
- abstracts - includes all sass helpers like variables, mixins, functions and placeholders that will not output css when compiled.
- base - includes reset styles, typography or boilerplate code which can be used to to all html elements.
- components -includes separate specific components like buttons, textbox, selection box, etc.
- layout - includes styles like header, footer, grids, navigation, etc.
- pages - includes specific styles for each page
- themes - theme styles if you implement themes in your project
- vendors - includes styles from other css frameworks like bootstrap.
Abstracts SCSS Folder
Under abstracts folder, let's create a new file _variables.scss and let's define our site colors and font. Copy the code below and past inside that file.
Under main.scss, import _variables.scss file so that it will be included during sass compilation. Remove all the code in main.scss file and copy the code below. Notice that you don't need to add underscore at the beginning of the file and also the file extension. SASS will automatically detect that this is scss file.
Base SCSS Folder
Under base folder, let's create a new file _base.scss, _fonts.scss and _reset.scss. Let's add fonts and styles for body html element and use the variables we created under abstracts folder.
Under _fonts.scss, we can add different fonts styles here either using external links like Google fonts or from your local files. In this tutorial, we will be using Google fonts.
Under _reset.scss, we need to reset the box sizing of all elements so that the width will not be affected by padding or border. If you want to Open normalize cssnormalize css, you may do so.
We need to add these three files in order to be included during compilation.
Since we added these styles in my previous tutorial regarding CSS Module, we need to comment related styles to avoid duplicates.
Layout SCSS Folder
Under this folder, let's create a new file _grid.scss and define our container, row and column classes. Copy the code below and paste it inside _grid.scss file.
Since we have define a container class in our previous tutorial about Open CSS ModuleCSS Module, we need to comment or remove max-width style under layout.module.css Open https://github.com/alferjay/shootace-gatsbyjs/blob/css-module/src/component/layout/layout.module.css file so that our max with define in _grid.scss will work.
We can now use .container class we define in _grid.scss and add it under our header.js Open https://github.com/alferjay/shootace-gatsbyjs/blob/css-module/src/component/layout/header/header.js and footer.js Open https://github.com/alferjay/shootace-gatsbyjs/blob/css-module/src/component/layout/footer/footer.js component.
Create homepage hero image cover using SCSS
Now we are ready to create the hero image in our homepage Open https://ajscript.com/web/image/627-babf5fc9/homepage.jpg. Let's open index.js inside pages folder and copy the code below. Get the image here Open https://github.com/alferjay/shootace-gatsbyjs/blob/sass/src/images/hero.jpg.
Components SCSS Folder
Under this folder, we need to create two files for the two components needed - _hero.scss and _line.scss. Since the line will be needed several times, it is better to add it as a component.
Lastly, we need to create a new file _header.scss in our layout scss folder and make the header position as absolute so that the hero image will start at the top of the page.
Don't forget to import all these files to main.scss in order to work.
Congratulations! You're now able to install, setup SASS in Gatsby and able to create a simple hero image using SCSS. You should see similar output below.
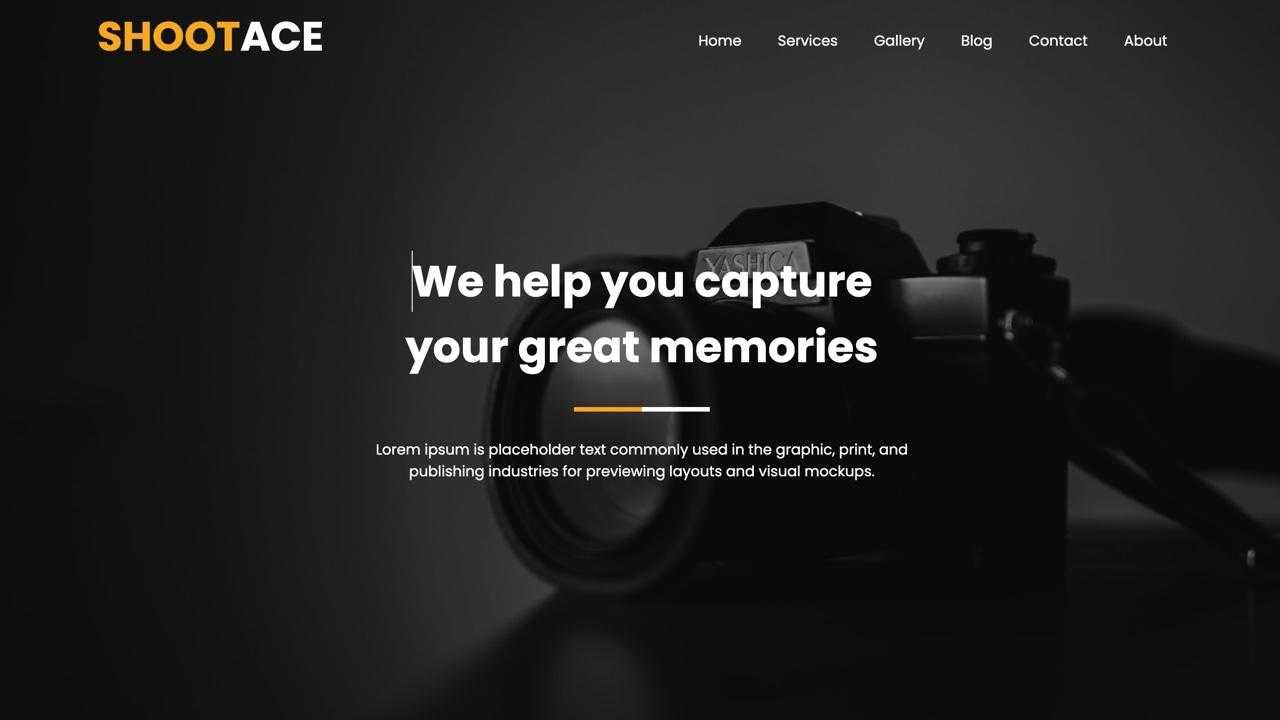